FREmu Documentation
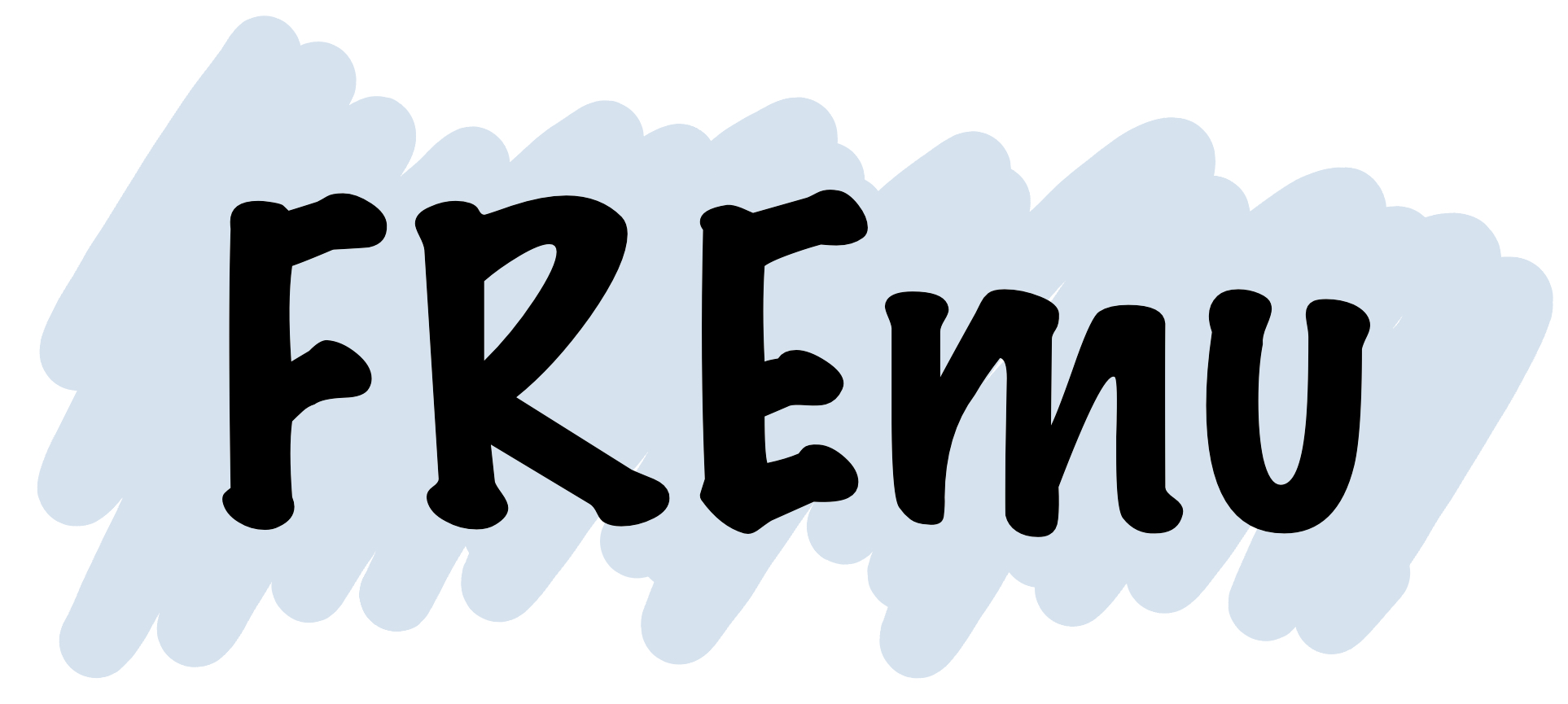
Overview
FREmu is designed to predict the non-linear power spectrum of large-scale structures in the universe using neural networks. It provides functionalities to set cosmological parameters, retrieve power spectra, boost factors, and error estimates.
Installation
pip install fremu
Usage
from fremu import fremu
# Initialize the emulator
emu = fremu.emulator()
Set Cosmological Parameters
# Set cosmological parameters
emu.set_cosmo(Om=0.3, Ob=0.05, h=0.7, ns=1.0, sigma8=0.8, mnu=0.05, fR0=-3e-5)
Get Power Spectrum
# Get power spectrum at redshift z and wave numbers k
power_spectrum = emu.get_power_spectrum(k=k_values, z=0.5)
Get Boost
# Get boost factor at redshift z and wave numbers k
boost_factor = emu.get_boost(k=k_values, z=0.5)
Get Error Estimate
# Get error estimate at wave number k (experimental)
error_estimate = emu.get_error(k=0.1)
Methods: emulator
get_k_values()
Get the wavenumbers used in the emulator.
- Returns: The array of wavenumbers.
set_cosmo(Om=0.3, Ob=0.05, h=0.7, ns=1.0, sigma8=0.8, mnu=0.05, fR0=-3e-5, redshifts=[3.0,2.0,1.0,0.5,0.0])
Set the cosmological parameters for the emulator.
Om
(float, optional): Matter density parameter Ωm. Default is 0.3.Ob
(float, optional): Baryon density parameter Ωb. Default is 0.05.h
(float, optional): Hubble constant H0 in units of 100 km/s/Mpc. Default is 0.7.ns
(float, optional): Scalar spectral index. Default is 1.0.sigma8
(float, optional): RMS matter fluctuations in spheres of radius 8 Mpc/h. Default is 0.8.mnu
(float, optional): Sum of neutrino masses in eV. Default is 0.05.fR0
(float, optional): Modified gravity parameter. Default is -3e-5.redshifts
(list of floats, optional): Redshift values to compute the matter power spectrum at. Default is [3.0,2.0,1.0,0.5,0.0].
get_boost(k=None, z=None, to_linear=False, return_k_values=False)
Get the boost factor for given wavenumbers and redshift.
k
(array_like, optional): The wavenumbers. Default is None, which uses the wavenumbers defined in the emulator.z
(float, optional): The redshift. Default is None, which uses z=0.0.to_linear
(bool, optional): Whether to convert the boost to linear power spectrum. Default is False.return_k_values
(bool, optional): Whether to return the wavenumbers along with the boost values. Default is False.- Returns: The boost factor at specified wavenumbers and redshift, or the wavenumbers along with the boost values if
return_k_values
is True.
get_power_spectrum(k=None, z=None, return_k_values=False, get_fid=False)
Get the power spectrum for given wavenumbers and redshift.
k
(array_like, optional): The wavenumbers. Default is None, which uses the wavenumbers defined in the emulator.z
(float, optional): The redshift. Default is None, which uses z=0.0.return_k_values
(bool, optional): Whether to return the wavenumbers along with the power spectrum values. Default is False.get_fid
(bool, optional): Whether to return the fiducial power spectrum. Default is False.- Returns: The power spectrum at specified wavenumbers and redshift, or the wavenumbers along with the power spectrum values if
return_k_values
is True. Ifget_fid
is True, returns the fiducial power spectrum.
get_error(k=None)
Get the error for given wavenumbers (experimental).
k
(array_like, optional): The wavenumbers. Default is None, which returns the entire error array.- Returns: The error at specified wavenumbers, or the entire error array if
k
is None.
Notes
- Ensure that cosmological parameters are set before retrieving power spectra or boost factors.